I searched and searched, and I could not find a way to model complex 3D parametric surfaces from an equation. For those who don’t know, 3D parametric surfaces are defined by a vector that traces a shape. Some examples are:
- boy’s knot
- breather
- mobius strip
- klein bottle
My solution was to use CSG (constructive solid geometry) software to effectively trace a small sphere at each point on the 3D parametric equation, taking infinitesimally small shapes. Then I printed it!
For a faster solution, read the entry titled “3D Printing with Mathematica!”
Example:
This basic surface, a cyclide, was rendered on OpenSCAD.
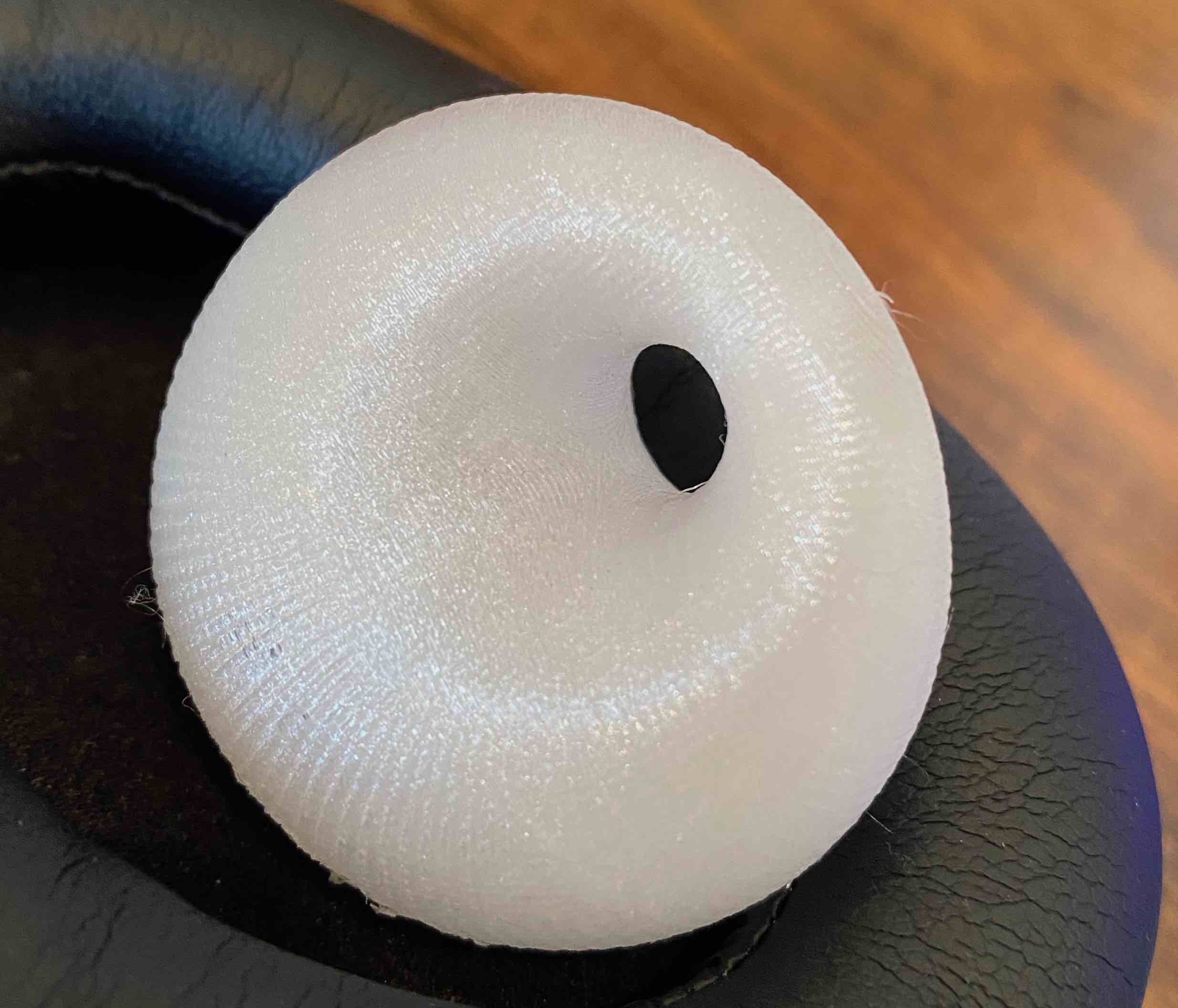
Basic Theory
This project existed with the goal of creating a simple universal script that could be used to simply and elegantly create a CAD drawing of any 3D parametric surface as long as one had an equation.
There are many mathematical surfaces that can be elegantly expressed as parametric equations. For example, take the implicit sphere equation:
$$x^2 + y^2 + z^2 = 30$$
By converting cartesian coordinates into spherical coordinates, we can arrive at the explicit equation for the spherical surface:
$$\vec{r}(\theta, \psi) = \sqrt{30}\sin{\psi}\cos{\theta}\vec{i} + \sqrt{30}\sin{\psi}\sin{\theta}\vec{j} + \sqrt{30}\cos{\theta}\vec{k}$$ where $$0 \leq \psi \leq \pi ; \text{and} ; 0\leq \theta \leq 2\pi$$
If we use a computer program to trace this vector over the intervals, and we place a small point on the coordinate plane for each “iteration” of these intervals, we can arrive at the outline of a sphere that is depicted by a point cloud. As we approach the limit of 0 for incrementation size, we increase the resolution of the point cloud, and these points begin to come together as one solid shape that can be elegantly described by the equation.
In Practice
I created a working version of this idea in the CSG modeling program OpenSCAD. OpenSCAD works by performing boolean operations (i.e. adding or subtracting) together very simple geometric shapes, such as spheres, cylinders, and rectangles, to create increasingly complex geometric shapes. For example, an image taken from their website demonstrating the use of their software.
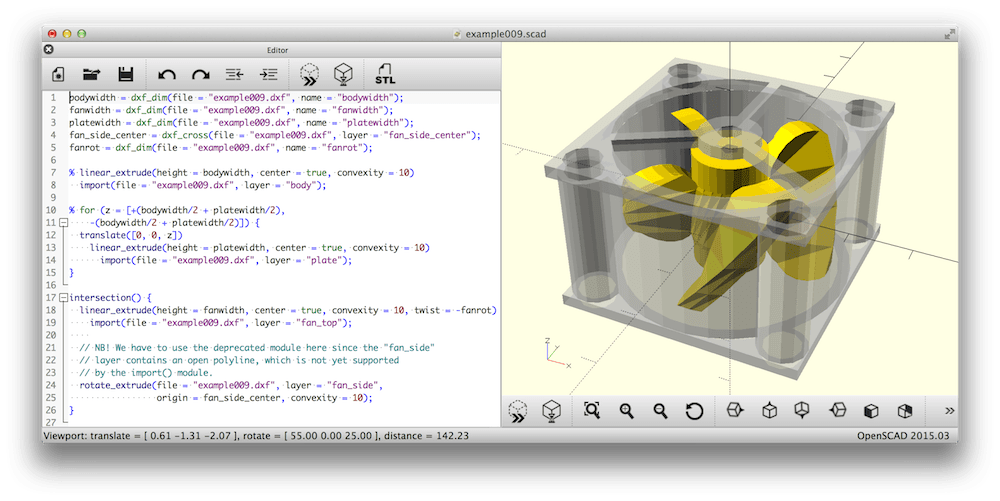
The script I wrote is quite straightforward. A for loop places small spheres along the path traced by the vector, r. As the amount of increments approaches infinity, these “dots” will become so packed that it becomes impossible to tell where one begins and the next one ends. The computer performs a boolean add operation on all of these dots, and the resulting geometry can be exported to an STL file for printing.
Example code for a Cyclide
module dupin_cyclide(step, size) {
for(u=[0:step:360]){
for(v=[0:step:360]){
/* cylinder
x = r;
y = 5*sin(theta);
z = 5*cos(theta);
//Takes 5 hours to render with a step size of 3
*/
mu = 8;
a = 13;
b = 12;
c = sqrt(a*a-b*b);
x = (mu*(c-a*cos(u)*cos(v))+b*b*cos(u))/(a-c*cos(u)*cos(v));
y = (b*(a-mu*cos(v))*sin(u))/(a-c*cos(u)*cos(v));
z = (b*(c*cos(u)-mu)*sin(v))/(a-c*cos(u)*cos(v));
translate( [x, y, z] ) sphere(size);
}
}
}
And such is an example of the output. OpenSCAD uses a very precise but slow single threaded algorithm (CGAL) and thus rendering this cyclide into an STL takes about 6 hours on my desktop. The STL file was absolutely huge at 250 megabytes because CGAL was computing infinitesimally small geometries.
Other Examples:
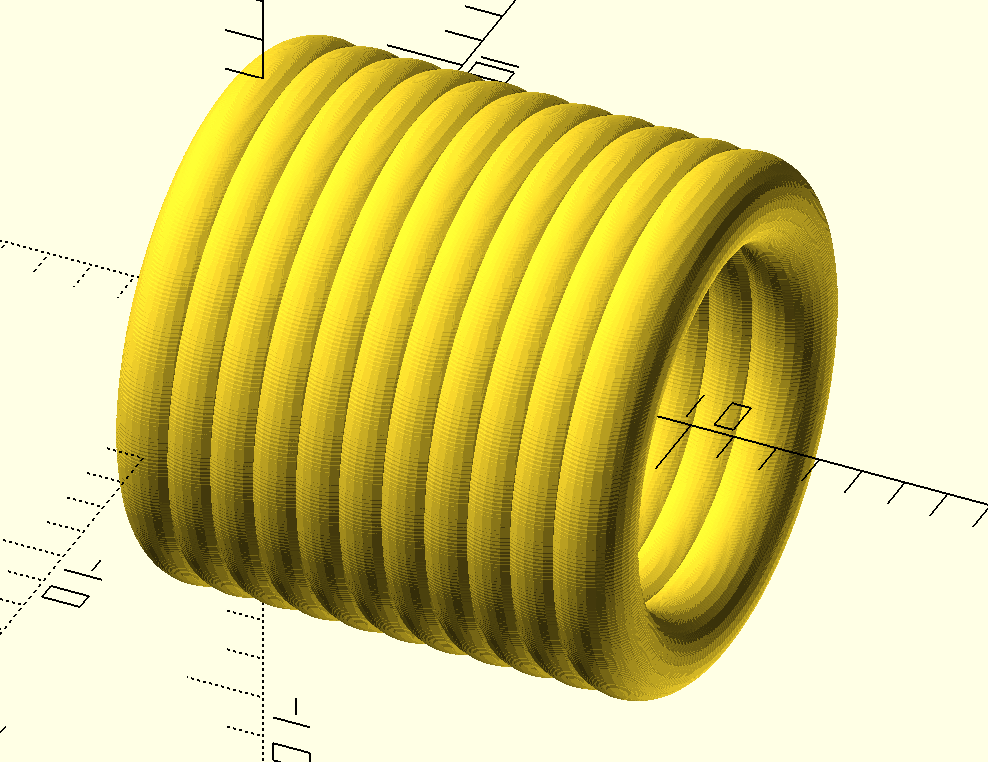
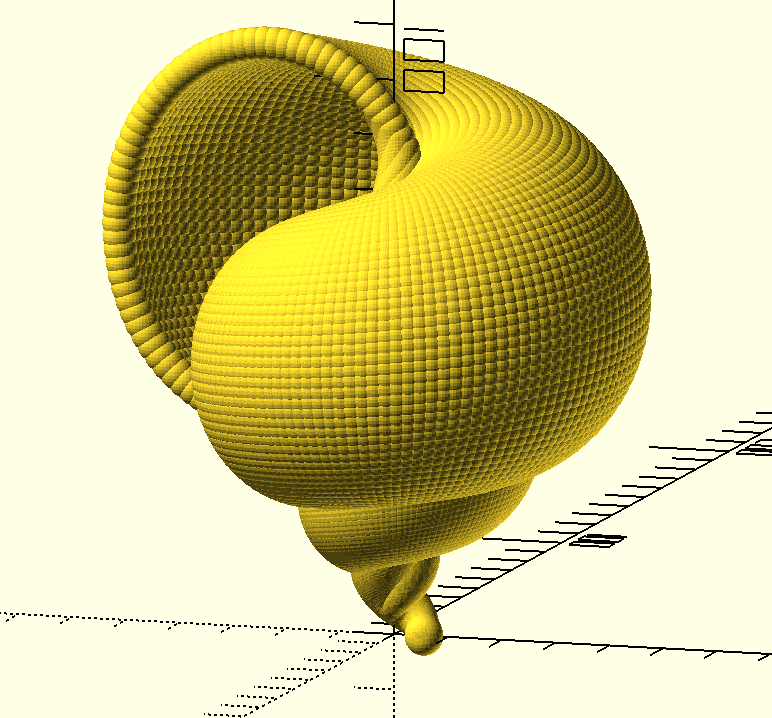
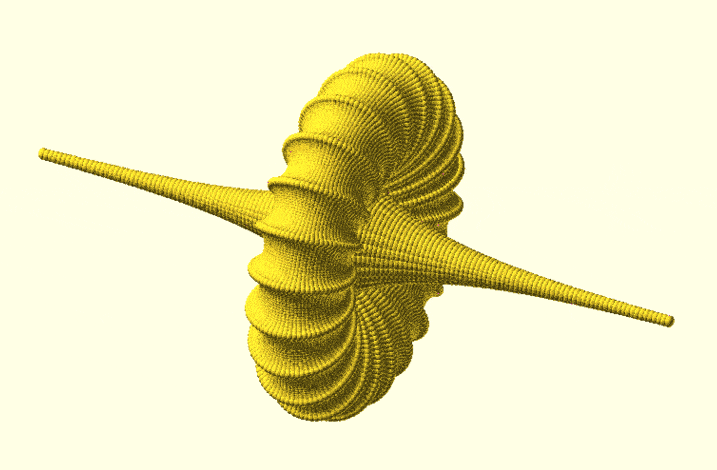
There are so many more cool looking surfaces out there that could be easily implemented into this script. However, I was quite disappointed in the computation time necessary for such a seemingly simple script.
I encourage you to spend a couple seconds checking out the 3D surface gallery that I have linked in the references section! There are many more common mathematical surfaces than just the ones that I have shown you. My favorites are the Klein Bottle, Boy’s Surface and Breather Surface.