Rendering Conway’s Game of Life in Blender
- Song: Comadza - Syntony
- Code was written in a couple hundred lines of Python posted here.
- Stills on my Artstation.
Intro
Blender is really cool software. Using Cycles (the provided raytracing engine), you can create really, really cool effects. Especially if you have an eye for proper lighting and shaders. Transparency and Volumetric effects look especially good, the way they catch light is absolutely fabulous, and the fact that we can do this all in a simulation is amazing.
This project took about 2 months to render on my computer (in 1440p). I have a 3080Ti and a 2060S, and I used both GPUs for the render. There was no greater meaning for the organisms I chose to display, I just chose whatever looked cool from an online lexicon.
Unfortunately because of youtube’s bitrate limitation, we get slight compression artifacts. I’ve included some 1440p stills that could be really nice wallpapers on my Artstation (see above).
Using Convolution Logic
Most people who are familiar with the logic of Conway’s game of life know that the program is written as instructions given a set of simple rules (from Wikipedia):
1. Any live cell with fewer than two live neighbors dies, as if by underpopulation.
2. Any live cell with two or three live neighbors lives on to the next generation.
3. Any live cell with more than three live neighbors dies, as if by overpopulation.
4. Any dead cell with exactly three live neighbors becomes a live cell, as if by reproduction.
Most implementations I’ve seen iterate over the grid in a loop and hardcode in these rules manually.
What many people don’t consider, however, is that conway’s game of life can also be expressed as a 2D convolution problem.
This translates to just a couple lines in Python!
# excerpt from the code
kernel = np.array([[1, 1, 1], [1, 0, 1], [1, 1, 1]], dtype=np.uint8)
def calculate_next(self):
C = convolve(self.grid, GameOfLife.kernel, mode='same')
next_grid = (
((self.grid == 1) & (C > 1) & (C < 4))
| ((self.grid == 0) & (C == 3))
).astype(np.uint8)
return next_grid
The code works first to calculate the number of neighbors of each cell in the grid using a convolution with the specified kernel. No loop!
Then, it applies the rules of the game of life to each cell in the matrix using a couple vectorized numpy operations! Nice!
Adapted from mikelane on Github.
Some Gifs
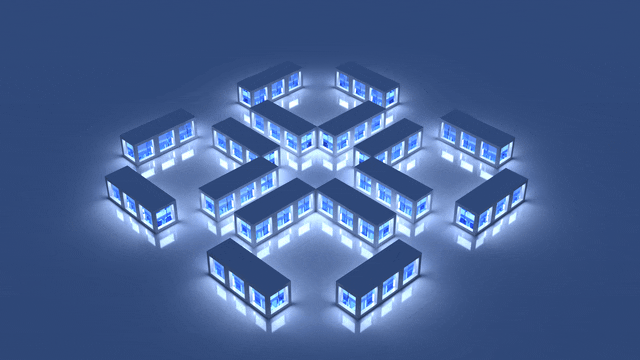
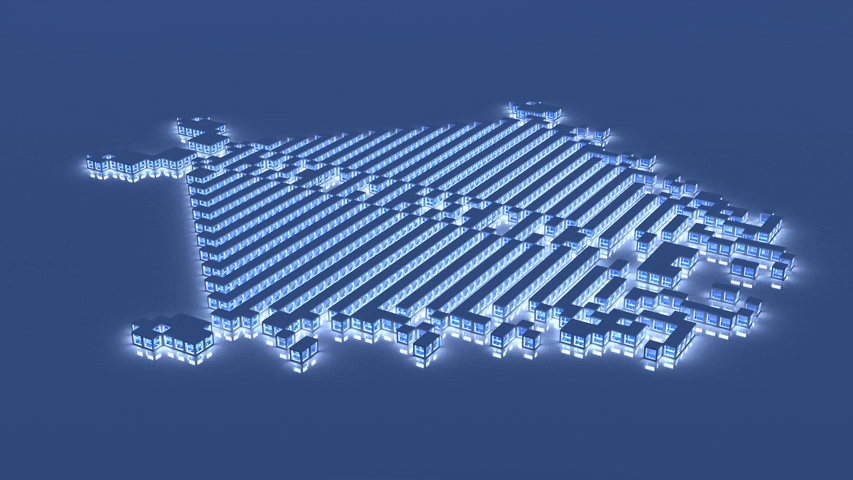
About John Conway
Sadly, John Conway died very recently in 2020 – he was really interesting to listen to in interviews.
Interestingly, he doesn’t see Game of Life as his greatest discovery, and wishes he was known for his other work!
“I don’t love it” - John Conway